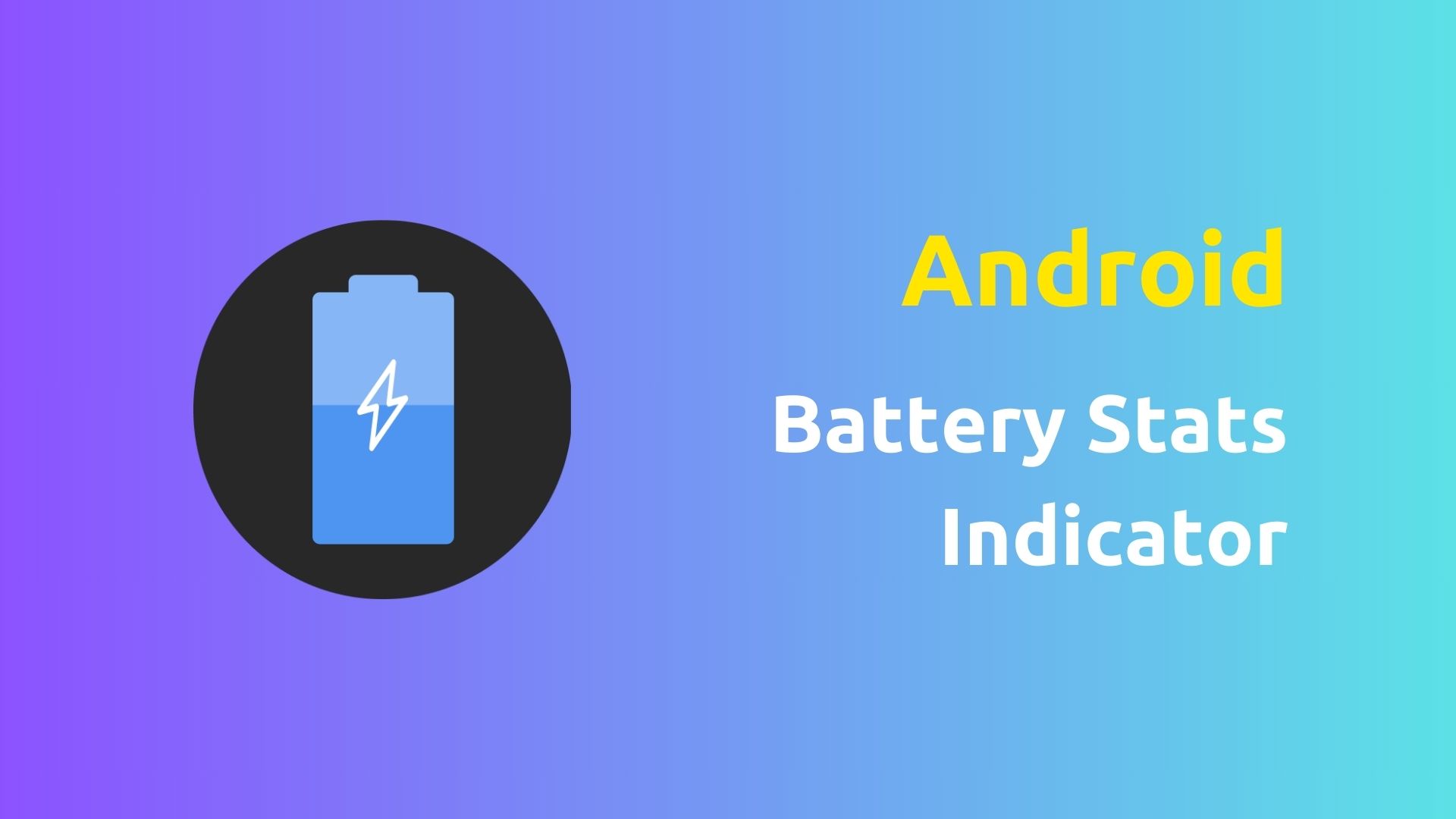
Simplify Battery Status Representation in Android Apps with Android Battery View Library
Managing battery status representation in Android apps can often be a time-consuming task for developers. It involves creating custom views, handling various states, and ensuring a visually appealing user experience. However, with the Android Battery View (ABV) library, developers can streamline this process, saving time and effort while enhancing their app's user interface.
The Android Battery View (ABV) library offers a simple yet powerful solution for integrating customizable battery views into Android apps. Born out of frustration with repetitive tasks, this lightweight library aims to simplify the process of displaying battery status, thereby improving the overall user experience.
Key Features:
- Lightweight: The ABV library is designed to be lightweight, ensuring minimal impact on app performance.
- Highly Customizable: Developers can customize various aspects of the battery view, including background colors, level colors, icons, size, and orientation.
- Kotlin and Java Support: The library supports both Kotlin and Java, making it accessible to a wide range of developers.
- BroadcastReceiver Integration: One of the unique features of ABV is its ability to automatically update battery status based on BroadcastReceiver intents, reducing the need for manual updates.
Demo
Normal | Charging | Warning | Critical |
---|---|---|---|
![]() | ![]() | ![]() | ![]() |
Prerequisites
Kotlin DSL
dependencyResolutionManagement {
repositoriesMode.set(RepositoriesMode.FAIL_ON_PROJECT_REPOS)
repositories {
google()
mavenCentral()
maven{
url = uri("https://jitpack.io")
}
}
}
Groovy DSL
dependencyResolutionManagement {
repositoriesMode.set(RepositoriesMode.FAIL_ON_PROJECT_REPOS)
repositories {
mavenCentral()
maven { url 'https://jitpack.io' }
}
}
Dependency
Kotlin DSL
dependencies {
...
implementation("com.github.ahmmedrejowan:AndroidBatteryView:0.1")
}
Groovy DSL
dependencies {
...
implementation 'com.github.ahmmedrejowan:AndroidBatteryView:0.1'
}
Usage
XML
<com.rejowan.abv.ABV
android:id="@+id/abv"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
app:abvBatteryOrientation="portrait"
app:abvRadius="10"
app:abvSize="50"/>
Kotlin
val abv = binding.abv
abv.size = 50
abv.mRadius = 10f
abv.chargeLevel = 50
abv.batteyOrientation = BatteryOrientation.PORTRAIT
abv.isCharging = false
BroadcastReceiver
This is the most unique feature of the library. You can attach your broadcast receiver intent to this view and it'll automatically show the status of the device battery.
- Create a broadcast receiver variable.
- Register the receiver to the activity/fragment
- Unregister when destroyed
- Attach the intent from the receiver to the view
Benefits
- You don't need to pass the charging level, status individually
- Automatically it'll get the charging level, stauts from the intent
- Minimal code with no issues
Here is an example
class MainActivity : AppCompatActivity() {
private val binding: ActivityMainBinding by lazy {ActivityMainBinding.inflate(layoutInflater)}
private val batteryReceiver: BroadcastReceiver = object : BroadcastReceiver() {
override fun onReceive(context: Context?, intent: Intent?) {
if (intent!=null){
binding.abv.attachBatteryIntent(intent)
}
}
}
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(binding.root)
registerReceiver(batteryReceiver, IntentFilter(Intent.ACTION_BATTERY_CHANGED))
}
override fun onDestroy() {
super.onDestroy()
unregisterReceiver(batteryReceiver)
}
}
Full Customization
XML
<com.rejowan.abv.ABV
android:id="@+id/abv"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_centerInParent="true"
app:abvNormalBgColor="#86B6F6"
app:abvNormalLevelColor="#4E94F1"
app:abvWarningBgColor="#FFCF96"
app:abvWarningLevelColor="#F5AD56"
app:abvCriticalBgColor="#EF5350"
app:abvCriticalLevelColor="#B71C1C"
app:abvChargingBgColor="#89EC9E"
app:abvChargingLevelColor="#4DD86C"
app:abvChargeLevel="5"
app:abvWarningChargeLevel="30"
app:abvCriticalChargeLevel="10"
app:abvIsCharging="false"
app:abvRadius="10"
app:abvBatteryOrientation="portrait"
app:abvSize="50"
app:abvChargingIcon="@drawable/ic_charge_abv"
app:abvWarningIcon="@drawable/ic_warning_abv" />
Kotlin
val abv = binding.abv
abv.normalBackgroundColor = ContextCompat.getColor(this, R.color.yourColor)
abv.normalLevelColor = ContextCompat.getColor(this, R.color.yourColor)
abv.warningBackgroundColor = ContextCompat.getColor(this, R.color.yourColor)
abv.warningLevelColor = ContextCompat.getColor(this, R.color.yourColor)
abv.criticalBackgroundColor = ContextCompat.getColor(this, R.color.yourColor)
abv.criticalLevelColor = ContextCompat.getColor(this, R.color.yourColor)
abv.chargingBackgroundColor = ContextCompat.getColor(this, R.color.yourColor)
abv.chargingLevelColor = ContextCompat.getColor(this, R.color.yourColor)
abv.chargingIcon = R.drawable.ic_charge_abv
abv.warningIcon = R.drawable.ic_warning_abv
abv.size = 50
abv.mRadius = 10f
abv.chargeLevel = 50
abv.warningChargeLevel = 30
abv.criticalChargeLevel = 10
abv.batteyOrientation = BatteryOrientation.PORTRAIT
abv.isCharging = false
Attribute
Full list of attributes available
Attribute | Format | Description |
---|---|---|
abvNormalBgColor | color | Background color for normal state |
abvNormalLevelColor | color | Level color for normal state |
abvWarningBgColor | color | Background color for warning state |
abvWarningLevelColor | color | Level color for warning state |
abvCriticalBgColor | color | Background color for critical state |
abvCriticalLevelColor | color | Level color for critical state |
abvChargingBgColor | color | Background color for charging state |
abvChargingLevelColor | color | Level color for charging state |
abvChargeLevel | integer | Level of charge |
abvWarningChargeLevel | integer | Warning level of charge |
abvCriticalChargeLevel | integer | Critical level of charge |
abvIsCharging | boolean | Indicates whether the device is charging |
abvRadius | integer | Radius of the battery view |
abvBatteryOrientation | enum | Orientation of the battery view |
abvSize | integer | Size of the battery view |
abvChargingIcon | reference | Icon displayed when charging |
abvWarningIcon | reference | Icon displayed in warning state |
Notes
- The library is in its early stages, so there may be some bugs.
- If you find any bugs, please report them in the
Issues
tab. - For Documentation, please visit the Documentation
- Sample app is available in the app directory.
Repository Link
The Android Battery View (ABV) library simplifies the process of integrating battery status representation into Android apps. By providing a lightweight and highly customizable solution, ABV helps developers save time and effort while enhancing the user experience. Whether you're building a simple utility app or a complex mobile application, ABV can be a valuable addition to your development toolkit.